Published by orzz.org(). (http://orzz.org/c%e8%ae%be%e8%ae%a1%e6%a8%a1%e5%bc%8f%e4%b9%8badapter%e9%80%82%e9%85%8d%e5%99%a8%e6%a8%a1%e5%bc%8f/)
很多时候,我们需要对一个已有的类做接口上的调整,如新的业务需要对已有的业务进行组合,或者新的模块需要使用现有的功能却不接收现有接口的调用形式...
在这种时候,我们需要有种可以将两套完全不同的接口标准统一的方法.适配器模式就是用来统一两套接口标准的一种模式.
适配器模式一般来说有两种实现方式,他们的类图分别如下:
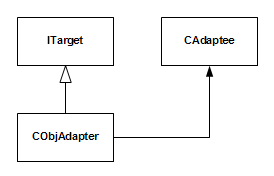
对象适配器模式类图

类适配器模式类图
这两种不同的适配器模式有着各自的适用范围.类适配器写起来方便,对象适配器则耦合更松.
下面是这两种适配器的C++示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 |
////////////////////////////////////////////////////////////////// // 适配器模式 C++示例 // // Author: 木头云 // Blog: http://darkc.at // E-Mail: memleak@darkc.at // Version: 1.0.819.1118(2009/08/19) ////////////////////////////////////////////////////////////////// #include "stdafx.h" ////////////////////////////////////////////////////////////////// // 目标接口类 class ITarget abstract { public: virtual ~ITarget() { } public: virtual void Request() = 0; }; // 需要被适配的类 class CAdaptee { public: void SpecificRequest() { _tcout << _T("特殊请求") << endl; } }; ////////////////////////////////////////////////////////////////// // 适配器类(对象适配器) class CObjAdapter : public ITarget { private: TSmartPtr<CAdaptee> adaptee; public: CObjAdapter() : adaptee(new CAdaptee) { } public: virtual void Request() { adaptee->SpecificRequest(); } }; // 适配器类(类适配器) class CClsAdapter : public ITarget, public CAdaptee { public: virtual void Request() { this->SpecificRequest(); } }; ////////////////////////////////////////////////////////////////// // 主函数 int _tmain(int argc, _TCHAR* argv[]) { TSmartPtr<ITarget> target; _tcout << _T("对象适配器:") << endl; // 对象适配器 target = new CObjAdapter; target->Request(); // 调用请求 _tcout << _T("类适配器:") << endl; // 类适配器 target = new CClsAdapter; target->Request(); // 调用请求 return 0; } ////////////////////////////////////////////////////////////////// /* 在软件系统中,由于应用环境的变化,常常需要将"一些现存的对象"放在新的环境中应用,但是新环境要求的接口是这些现存对象所不满足的. Adapter模式将一个类的接口转换成客户希望的另外一个接口,使得原本由于接口不兼容而不能一起工作的那些类可以一起工作. Adapter模式有对象适配器和类适配器两种形式的实现结构,但是类适配器采用"多继承"的实现方式.带来了不良的高耦合.所以一般不推荐使用. 对象适配器采用"对象组合"的方式.更符合松耦合精神. Adapter模式本身要求我们尽可能地使用"面向接口的编程"风格,这样才能在后期很方便的适配. */ ////////////////////////////////////////////////////////////////// |
代码运行结果如下:
1 2 3 4 5 |
对象适配器: 特殊请求 类适配器: 特殊请求 请按任意键继续. . . |